XMLHttpRequest Interview Preparation Guide Download PDF
XMLHttpRequest related Frequently Asked Questions in various XHR2 job interviews by interviewer. The set of questions here ensures that you offer a perfect answer posed to you. So get preparation for your new job hunting
28 XHR2 Questions and Answers:
Table of Contents
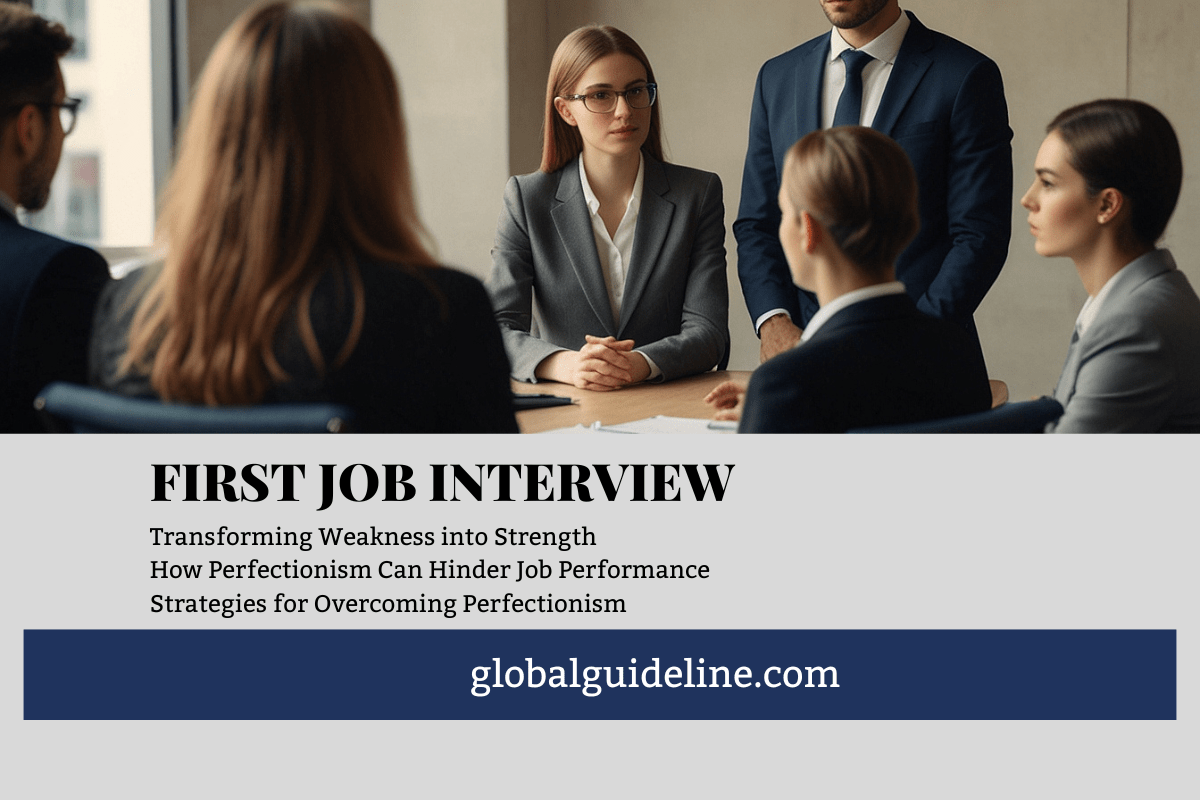
1 :: What is responseText?
The 'responseText' property is a string version of data returned from server process.
2 :: What is onreadystatechange?
The 'onreadystatechange' property fires at every state change event.
3 :: What is readyState?
The 'readyState' property is an object status integer. It uses the integers 0 to 4 for uninitialized, loading, loaded, interactive and complete states.
4 :: What is getAllResponseHeaders()?
The getAllResponseHeaders() method is used to return the full set of headers as a string.
5 :: What is status?
The 'status' property is for returning numeric codes from the server like error codes, etc.
6 :: Explain setRequestHeader("label", "value")?
The setRequestHeader("label", "value") method is used to assign a label/value pair to the header to be sent with a request.
7 :: How do we pass parameters to the server?
Below are the two ways of passing data to server. The first one shows by using GET and the second by POST.
xmlHttpObj.open("GET","http://" + location.host +
"/XmlHttpExample1/WebForm1.aspx?value=123", true);
xmlHttpObj.open("POST","http://" + location.host +
"/XmlHttpExample1/WebForm1.aspx?value=123", true);
xmlHttpObj.open("GET","http://" + location.host +
"/XmlHttpExample1/WebForm1.aspx?value=123", true);
xmlHttpObj.open("POST","http://" + location.host +
"/XmlHttpExample1/WebForm1.aspx?value=123", true);
8 :: Explain the purpose of each of the HTTP request types when used with a RESTful web service?
The purpose of each of the HTTP request types when used with a RESTful web service is as follows:
► GET: Retrieves data from the server (should only retrieve data and should have no other effect).
► POST: Sends data to the server for a new entity. It is often used when uploading a file or submitting a completed web form.
► PUT: Similar to POST, but used to replace an existing entity.
► PATCH: Similar to PUT, but used to update only certain fields within an existing entity.
► DELETE: Removes data from the server.
► TRACE: Provides a means to test what a machine along the network path receives when a request is made. As such, it simply returns what was sent.
► OPTIONS: Allows a client to request information about the request methods supported by a service. The relevant response header is Allow and it simply lists the supported methods. (It can also be used to request information about the request methods supported for the server where the service resides by using a * wildcard in the URI.)
► HEAD: Same as the GET method for a resource, but returns only the response headers (i.e., with no entity-body).
► CONNECT: Primarily used to establish a network connection to a resource (usually via some proxy that can be requested to forward an HTTP request as TCP and maintain the connection). Once established, the response sends a 200 status code and a "Connection Established" message.
► GET: Retrieves data from the server (should only retrieve data and should have no other effect).
► POST: Sends data to the server for a new entity. It is often used when uploading a file or submitting a completed web form.
► PUT: Similar to POST, but used to replace an existing entity.
► PATCH: Similar to PUT, but used to update only certain fields within an existing entity.
► DELETE: Removes data from the server.
► TRACE: Provides a means to test what a machine along the network path receives when a request is made. As such, it simply returns what was sent.
► OPTIONS: Allows a client to request information about the request methods supported by a service. The relevant response header is Allow and it simply lists the supported methods. (It can also be used to request information about the request methods supported for the server where the service resides by using a * wildcard in the URI.)
► HEAD: Same as the GET method for a resource, but returns only the response headers (i.e., with no entity-body).
► CONNECT: Primarily used to establish a network connection to a resource (usually via some proxy that can be requested to forward an HTTP request as TCP and maintain the connection). Once established, the response sends a 200 status code and a "Connection Established" message.
9 :: Can you explain Scriptmanager control in Ajax?
Scriptmanager control is the central heart of Ajax. They manage all the Ajax related objects on the page. Some of the core objectives of scriptmanager control are as follows:-
► Helps load core Ajax related script and library.
► Provides access to web services.
► ASP.NET authentication, role and profile services are loaded by scriptmanager control.
► Provided registration of server controls and behaviors.
► Enable full or partial rendering of a web page.
► Provide localization features.
In short , any Ajax enable page should have this control.
► Helps load core Ajax related script and library.
► Provides access to web services.
► ASP.NET authentication, role and profile services are loaded by scriptmanager control.
► Provided registration of server controls and behaviors.
► Enable full or partial rendering of a web page.
► Provide localization features.
In short , any Ajax enable page should have this control.
10 :: Explain Open ("method", "URL", "async", "uname", "pswd")?
Open ("method", "URL", "async", "uname", "pswd"):- This method takes a URL and other values needed for a request. You can also specify how the request is sent by GET, POST, or PUT. One of the important values is how this request will be sent asynchronously or synchronously. True means that processing is carried after the send () method, without waiting for a response. False means that processing is waits for a response before continuing.
11 :: What is Ready State?
Ready State: - Returns the current state of the object.
0 = uninitialized
1 = loading
2 = loaded
3 = interactive
4 = complete
0 = uninitialized
1 = loading
2 = loaded
3 = interactive
4 = complete
12 :: How do I get the XMLHttpRequest object?
Depending upon the browser...
if (window.ActiveXObject) {
// Internet Explorer
http_request = new ActiveXObject("Microsoft.XMLHTTP");
}
else if...
if(window.XMLHttpRequest)
{
xmlhttpobj=new XMLHttpRequest();
return xmlhttpobj;
}
else
{
try
{
xmlhttpobj=new ActiveXObject("Microsoft.XMLHTTP");
}catch(e)
{
xmlhttpobj=new ActiveXObject("Msxml2.XMLHTTP");
}
}
}
if (window.ActiveXObject) {
// Internet Explorer
http_request = new ActiveXObject("Microsoft.XMLHTTP");
}
else if...
if(window.XMLHttpRequest)
{
xmlhttpobj=new XMLHttpRequest();
return xmlhttpobj;
}
else
{
try
{
xmlhttpobj=new ActiveXObject("Microsoft.XMLHTTP");
}catch(e)
{
xmlhttpobj=new ActiveXObject("Msxml2.XMLHTTP");
}
}
}
13 :: Explain what is JSON?
JSON is a very lightweight data format based on a subset of the JavaScript syntax, namely array and object literals. JSON allows communicating with server in a standard way. JSON is used as communication notation instead of XML.
Hide Copy Code
var oBike =
{
"color" : "Green",
"Speed": 200,
};
alert(oBike.color); //outputs "Green"
alert(oBike.Speed); //outputs 200
The above code creates an javascript object bike with two properties Color and Speed.
Hide Copy Code
var oBike =
{
"color" : "Green",
"Speed": 200,
};
alert(oBike.color); //outputs "Green"
alert(oBike.Speed); //outputs 200
The above code creates an javascript object bike with two properties Color and Speed.
14 :: What is CORS? How does it work?
Cross-origin resource sharing (CORS) is a mechanism that allows many resources (e.g., fonts, JavaScript, etc.) on a web page to be requested from another domain outside the domain from which the resource originated. It's a mechanism supported in HTML5 that manages XMLHttpRequest access to a domain different.
CORS adds new HTTP headers that provide access to permitted origin domains. For HTTP methods other than GET (or POST with certain MIME types), the specification mandates that browsers first use an HTTP OPTIONS request header to solicit a list of supported (and available) methods from the server. The actual request can then be submitted. Servers can also notify clients whether "credentials" (including Cookies and HTTP Authentication data) should be sent with requests.
CORS adds new HTTP headers that provide access to permitted origin domains. For HTTP methods other than GET (or POST with certain MIME types), the specification mandates that browsers first use an HTTP OPTIONS request header to solicit a list of supported (and available) methods from the server. The actual request can then be submitted. Servers can also notify clients whether "credentials" (including Cookies and HTTP Authentication data) should be sent with requests.
15 :: What is the fundamental behind Ajax?
XmlHttpRequest is the fundamental behind Ajax. This allows the browser to communicate to a back end server asynchronously.XmlHttpRequest object allows the browser to communicate with server with out posting the whole page and only sending the necessary data asynchronously
16 :: How can we consume data directly in web services?
We can consume data directly using 'Sys.Data' controls. We know this is a very short answer for such an important question, but the bandwidth of this book does not allow for the same. We suggest the readers to practice some sample using the 'Sys.Data' control.
Note: - We have left important controls like Data controls, Login controls, Web part controls, mobile controls and profilescriptservice control. These controls will be rarely asked during interviews, but from project aspects they are very important.
Note: - We have left important controls like Data controls, Login controls, Web part controls, mobile controls and profilescriptservice control. These controls will be rarely asked during interviews, but from project aspects they are very important.
17 :: What is responseXML?
The 'responseXML' property is DOM-compatible document object of data returned from server process.
18 :: What is statusText?
The 'statusText' property is used for string messages that accompany the status code.
19 :: Explain abort() Function?
The abort() is used to stop the current request.
20 :: What is getResponseHeader("headerLabel")?
The getResponseHeader("headerLabel") method is used to return the string value of a single header label.
21 :: What is open(arg, arg,arg,arg,arg) function?
The open("method", "URL"[, asyncFlag[, "userName"[, "password"]]]) is used to assign the destination URL, method, and other optional attributes of a request.
22 :: What is send(content)?
The send(content) method transmits the request, optionally with postable string or the data of DOM object.
23 :: How do we do asynchronous processing using Ajax?
xmlHttpObj.onreadystatechange = function1();
Above is the code snippet, which will help us to do asynchronous processing. So function1 () will be called when the XMLHTTP request object goes to on ready state change.
Above is the code snippet, which will help us to do asynchronous processing. So function1 () will be called when the XMLHTTP request object goes to on ready state change.
24 :: What is Response Text?
Response Text: - Returns the response in plain string.
25 :: What is SetRequestHeader ("label"," value")?
SetRequestHeader ("label"," value"):- Sets label value pair for a HTTP header.