Web Development Ninjas Interview Preparation Guide Download PDF
Web Development Ninjas related Frequently Asked Questions in various Ninjas Web Developer job interviews by interviewer. The set of questions here ensures that you offer a perfect answer posed to you. So get preparation for your new job hunting
58 Ninjas Web Developer Questions and Answers:
Table of Contents
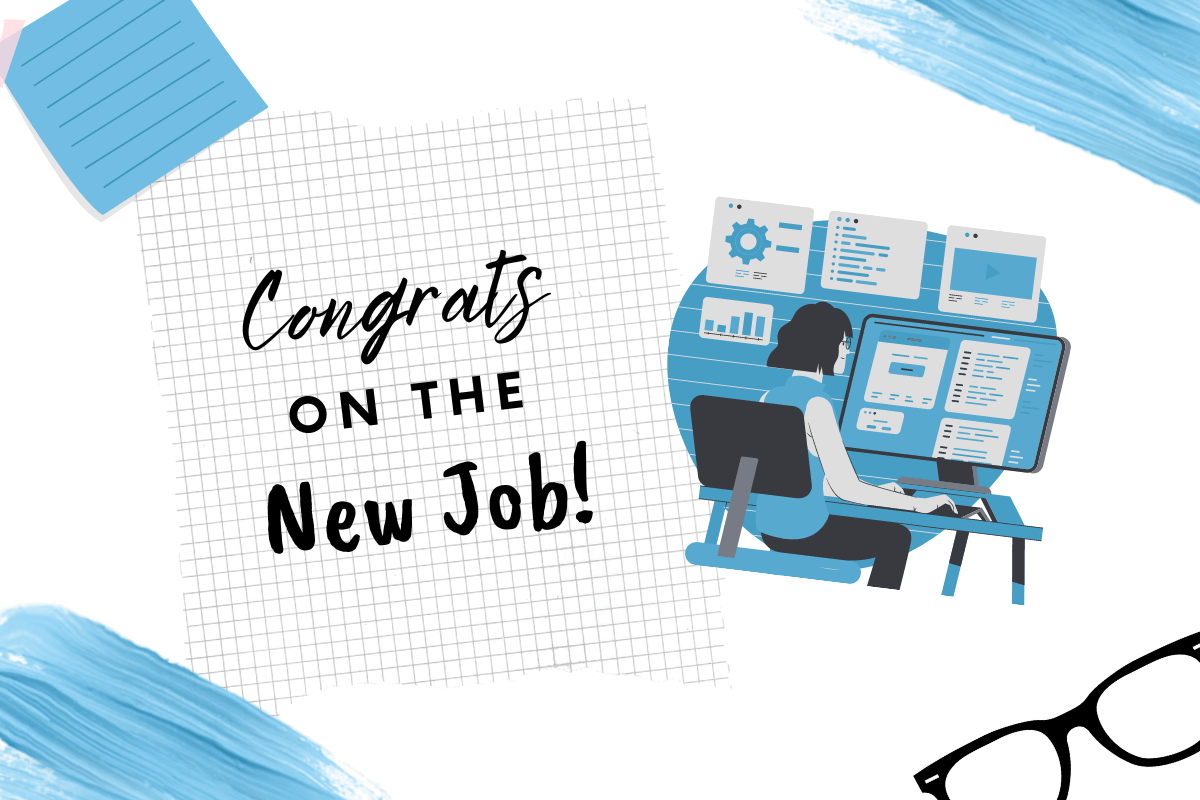
1 :: Explain me what are three ways to reduce page load time?
Reduce image sizes, remove unnecessary widgets, HTTP compression, put CSS at the top and script references at the bottom or in external files, reduce lookups, minimize redirects, caching, etc.
2 :: Please explain how do you make comments without text being picked up by the browser?
Comments are used to explain and clarify code or to prevent code from being recognized by the browser. Comments start with “*<!--” and end with ” -->“.
<!-- Insert comment here. -->
<!-- Insert comment here. -->
3 :: Do you know what are some new HTML5 markup elements?
There are several: <article>, <aside>, <bdi>, <command>, <details>, <figure>, <figcaption>, <summary>, <header>, <footer>, <hgroup>, <mark>, <meter>, <nav>, <progress>, <ruby>, <rt>, <section>, <time>, and <wpr>.
4 :: Tell me why did you decide to enter the world of gaming? How long have you been creating games?
I’ve always been an avid gamer going back to the late 80’s with the Nintendo (NES) system, then the Sega Mega Drive and Game Boy in the 90’s, then onto the Playstation. I’ve always wanted to make games but back then it was nearly impossible for an indie dev. That was until the invention
5 :: Tell me what is $() in jQuery library?
The $() function is an alias of jQuery() function, at first it looks weird and makes jQuery code cryptic, but once you get used to it, you will love it’s brevity. $() function is used to wrap any object into jQuery object, which then allows you to call various method defined jQuery object. You can even pass a selector string to $() function, and it will return jQuery object containing an array of all matched DOM elements. I have seen this jQuery asked several times, despite it’s quite basic, it is used to differentiate between developer who knows jQuery or not.
6 :: Explain me what is the each() function in jQuery? How do you use it?
each() function is like an Iterator in Java, it allows you to iterate over a set of elements. You can pass a function to each() method, which will be executed for each element from the jQuery object, on which it has been called. This question sometime is asked as a follow-up of the previous question e.g. how to show all selected options in alert box. We can use the above selector code to find all the selected options and then we can use the each() method to print them in an alert box, one by one, as shown below:
$('[name=NameOfSelectedTag] :selected').each(function(selected){
alert($(selected).text());
});
The text() method returns text for that option.
$('[name=NameOfSelectedTag] :selected').each(function(selected){
alert($(selected).text());
});
The text() method returns text for that option.
7 :: Tell me what is method chaining in jQuery? What is the benefit of using method chaining?
Method chaining is calling another method on the result of another method, it results in clean and concise code, single search over DOM so better performance.
8 :: Explain have you learned something new or interesting lately?
Make sure you know all the relevant news and blogs. You should be reading them regardless, but doing so on a daily basis during your job search is important. Be ready to talk casually and fluently about the latest web trends.
9 :: Tell me what is the difference between HTML elements and tags?
HTML elements communicate to the browser how to render text. When surrounded by angular brackets <> they form HTML tags. For the most part, tags come in pairs and surround text.
10 :: Tell me what is the difference between linking to an image, a website, and an email address?
o link an image, use <img> tags. You need specify the image in quotes using the source attribute, src in the opening tag. For hyperlinking, the anchor tag, <a>, is used and the link is specified in the href attribute. Text to be hyperlinked should be placed between the anchor tags. Little known fact: href stands for “hypertext reference.” When linking to an email, the href specification will be “mailto:send@here.com.” See examples below:
<img src=”HTMLLinks.jpg”></img>
<a href=”http://www.globalguideline.com/”>Global Guideline</a>
<a href=”hussain@globalguideline.com.com”>Email Me</a>
<img src=”HTMLLinks.jpg”></img>
<a href=”http://www.globalguideline.com/”>Global Guideline</a>
<a href=”hussain@globalguideline.com.com”>Email Me</a>
11 :: What is the new DOCTYPE?
Instead of typing out a ridiculously long DOCTYPE statement to tell the browser how to render your webpage, this long line of code has been truncated to <!doctype html>.
12 :: Do you know what are data- attributes good for?
The HTML5 data- attribute is a new addition that assigns custom data to an element. It was built to store sensitive or private data that is exclusive to a page or application, for which there are no other matching attributes or elements.
13 :: Explain me why did you decide to add multiplayer to your game? Why Nextpeer?
For me it’s a no-brainer – it’s more fun to play with others than playing by yourself. Multiplayer has been proven to improve retention within your games, to get players coming back for just one more try to beat their friends. It’s easy and cheap to integrate it – so why not?
What are the biggest challenges of a game developer? If you can, please tell us how you overcame some of those challenges?
At the beginning, the biggest challenge was price – it was hard to get games made for low cost. As the industry has evolved, source codes became readily available to purchase cheaply, modify and improve. The big challenge now is competition – the market is getting more crowded and it’s harder for a developer’s games to be found. You have to have your finger on the pulse of the market and be ready to adapt at a moment’s notice, as this business changes so quickly.
What are the biggest challenges of a game developer? If you can, please tell us how you overcame some of those challenges?
At the beginning, the biggest challenge was price – it was hard to get games made for low cost. As the industry has evolved, source codes became readily available to purchase cheaply, modify and improve. The big challenge now is competition – the market is getting more crowded and it’s harder for a developer’s games to be found. You have to have your finger on the pulse of the market and be ready to adapt at a moment’s notice, as this business changes so quickly.
14 :: Suppose you have five <div> element in your page? How do you select them using jQuery?
Another fundamental jQuery question based on selector. jQuery supports different kinds of selector e.g. ID selector, class selector and tag selector. Since in this question nothing has been mentioned about ID and class, you can use the tag selector to select all div elements. jQuery code : $(“div”), will return a jQuery object contain all five div tags. For more detailed answer, see the article.
15 :: Explain me difference between JavaScript window.onload event and jQuery ready function?
This is the follow-up of the previous question. The main difference between the JavaScript onload event and the jQuery ready function is that the former not only waits for DOM to be created but also waits until all external resources are fully loaded including heavy images, audios and videos. If loading images and media content takes lot of time, then the user might experience significant delay on the execution of code defined in the window.onload event.
On the other hand, the jQuery ready() function only waits for the DOM tree, and does not wait for images or external resource loading, something that means faster execution. Another advantage of using the jQuery $(document).ready() is that you can use it at multiple times in your page, and the browser will execute them in the order they appear in the HTML page, as opposed to the onload technique, which can only be used for a single function. Given this benefits, it’s always better to use the jQuery ready() function rather than the JavaScript window.onload event.
On the other hand, the jQuery ready() function only waits for the DOM tree, and does not wait for images or external resource loading, something that means faster execution. Another advantage of using the jQuery $(document).ready() is that you can use it at multiple times in your page, and the browser will execute them in the order they appear in the HTML page, as opposed to the onload technique, which can only be used for a single function. Given this benefits, it’s always better to use the jQuery ready() function rather than the JavaScript window.onload event.
16 :: Tell me how do you add and remove CSS classes to an element using jQuery?
By using the addClass() and removeClass() jQuery methods. This can be very handy, while dynamically changing class of elements e.g. marking them inactive or active and using class “.active” etc.
17 :: Tell me which one is more efficient: document.getElementbyId( “myId”) or $(“#myId)?
The first one because its a direct call to the JavaScript engine.
18 :: Tell me can you write jQuery code to select all links, which is inside paragraphs?
Another jQuery interview question based on selectors. This is also required to write a jQuery one liner, like many other questions. You can use the following jQuery snippet to select all links (<a> tag) nested inside paragraphs (<p> tag).
19 :: Explain me difference between ID selector and class selector in jQuery?
If you have used CSS, then you might know the difference between ID and class selector, It’s the same with jQuery. ID selector uses ID e.g. #element1 to select element, while class selector uses CSS class to select elements. When you just need to select only one element, use ID selector, while if you want to select a group of element, having same CSS class than use class selector. There is good chance that during the interview you will be asked to write code using ID and class selector. The following jQuery code uses ID and class selectors:
$('#LoginTextBox') -- Returns element wrapped as jQuery object with id='LoginTextBox'
$('.active') -- Returns all elements with CSS class active.
From a syntax perspective, as you can see, another difference between ID and class selector is that former uses “#” and later uses “.” character. More detailed analysis and discussion, see answer.
$('#LoginTextBox') -- Returns element wrapped as jQuery object with id='LoginTextBox'
$('.active') -- Returns all elements with CSS class active.
From a syntax perspective, as you can see, another difference between ID and class selector is that former uses “#” and later uses “.” character. More detailed analysis and discussion, see answer.
20 :: Tell me what are some of the major new API’s that come standard with HTML5?
To name a few: Media API, Text Track API, Application Cache API, User Interaction, Data Transfer API, Command API, Constraint Validation API, and the History API.
21 :: Tell me what is the difference between the application model of HTML and HTML5?
Trick question, there is no difference. HTML5 is a continuum of HTML and just a souped up version of the original HTML. There has been no major paradigm shift.
22 :: Do you know what does DOCTYPE mean?
The term DOCTYPE tells the browser which type of HTML is used on a webpage. In turn, the browsers use DOCTYPE to determine how to render a page. Failing to use DOCTYPE or using a wrong DOCTYPE may load your page in Quirks Mode. See example:
<!DOCTYPE HTML PUBLIC "-//W3C//DTD HTML 4.01 Transitional//EN" "http://www.w3.org/TR/html4/loose.dtd">.
<!DOCTYPE HTML PUBLIC "-//W3C//DTD HTML 4.01 Transitional//EN" "http://www.w3.org/TR/html4/loose.dtd">.
23 :: Tell us why did you get into coding, programming, etc.?
“Because I can make good $,” “I don’t like to dress up or shave,” and “because I loved the movie Hackers,” are not good enough answers. Well… a comment about Hackers might fly but make sure you have a real backstory that describes your “Aha!” moment.
24 :: Tell me how many HTML tags are should be used for the most simple of web pages?
8 total. 4 pairs of tags.
<HTML>
<HEAD>
<TITLE>Simplest page ever!</TITLE>
</HEAD>
<BODY>
Doesn’t get simpler than this.
</BODY>
</HTML>
<HTML>
<HEAD>
<TITLE>Simplest page ever!</TITLE>
</HEAD>
<BODY>
Doesn’t get simpler than this.
</BODY>
</HTML>
25 :: Explain me what are some new input attributes in HTML5?
There are many new form elements including: datalist, datetime, output, keygen, date, month, week, time, number, range, email, and url.