Jnr PHP/Codeigniter Developer Interview Preparation Guide Download PDF
Jnr PHP/Codeigniter Developer Frequently Asked Questions in various Jnr PHP/Codeigniter Developer job interviews by interviewer. The set of questions are here to ensures that you offer a perfect answer posed to you. So get preparation for your new job interview
61 Jnr PHP/Codeigniter Developer Questions and Answers:
Table of Contents
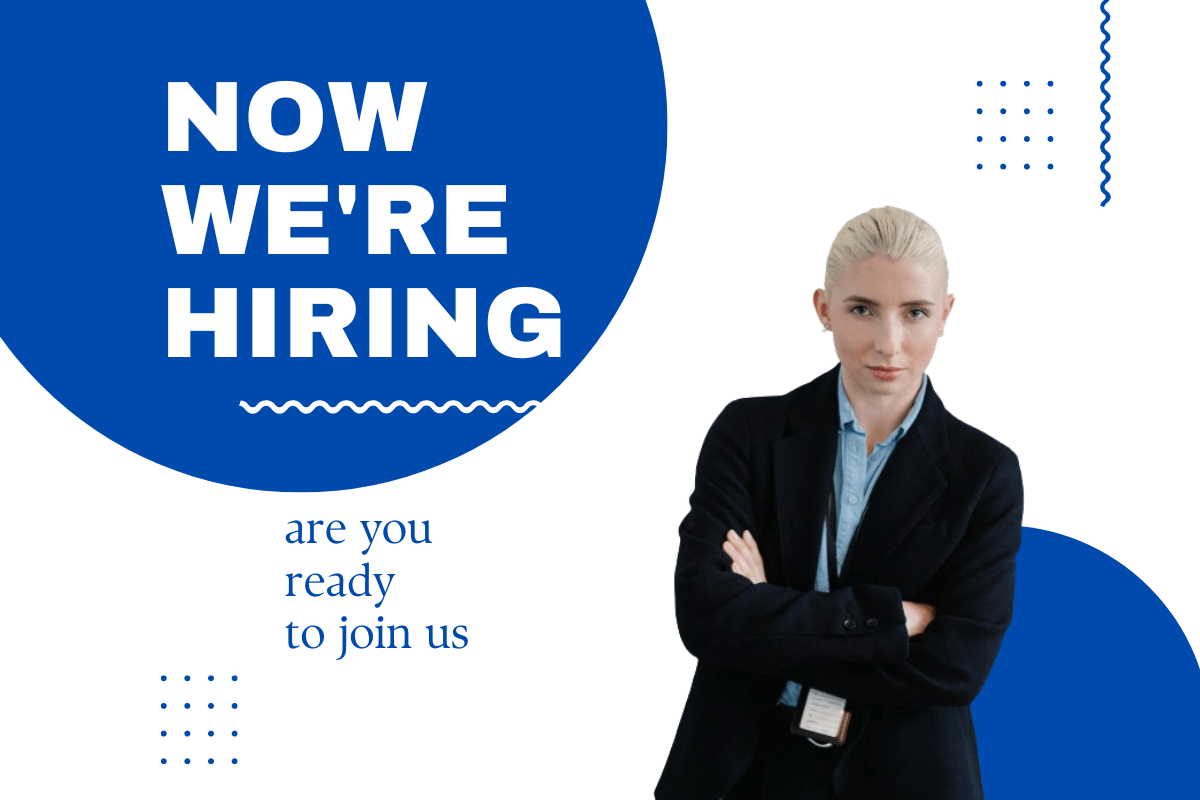
1 :: Tell us what helpers in CodeIgniter are and how you can load a helper file?
In CodeIgniter, helpers are group of function in a particular category that assist you to perform specific functions. In CodeIgniter, you will find many helpers like URL helpers- helping in creating links, Text helpers- perform various text formatting routines, Cookies- helpers set and read cookies. You can load helper file by using command $this->load->helper (‘name’) ;
2 :: Do you know what is inhibitor in CodeIgniter?
For CodeIgniter, inhibitor is an error handler class, using the native PHP functions like set_exception_handler, set_error_handler, register_shutdown_function to handle parse errors, exceptions, and fatal errors.
3 :: Tell me how you can enable CSRF (Cross Site Request Forgery) in CodeIgniter?
You can activate CSRF (Cross Site Request Forgery) protection in CodeIgniter by operating your application/config/config.php file and setting it to
$config [ ‘csrf_protection’] = TRUE;
If you avail the form helper, the form_open() function will insert a hidden csrf field in your forms automatically
$config [ ‘csrf_protection’] = TRUE;
If you avail the form helper, the form_open() function will insert a hidden csrf field in your forms automatically
4 :: Do you know how can you enable error reporting in PHP?
Check if “display_errors” is equal “on” in the php.ini or declare “ini_set('display_errors', 1)” in your script.
Then, include “error_reporting(E_ALL)” in your code to display all types of error messages during the script execution.
Enabling error messages is very important especially during the debugging process as one can instantly get the exact line that is producing the error and can see also if the script in general is behaving correctly.
Then, include “error_reporting(E_ALL)” in your code to display all types of error messages during the script execution.
Enabling error messages is very important especially during the debugging process as one can instantly get the exact line that is producing the error and can see also if the script in general is behaving correctly.
5 :: Explain me how would you declare a function that receives one parameter name hello?
If hello is true, then the function must print hello, but if the function doesn’t receive hello or hello is false the function must print bye.
<?php
function showMessage($hello=false){
echo ($hello)?'hello':'bye';
}
?>
<?php
function showMessage($hello=false){
echo ($hello)?'hello':'bye';
}
?>
6 :: Do you know what are SQL Injections, how do you prevent them and what are the best practices?
SQL injections are a method to alter a query in a SQL statement send to the database server. That modified query then might leak information like username/password combinations and can help the intruder to further compromise the server.
To prevent SQL injections, one should always check & escape all user input. In PHP, this is easily forgotten due to the easy access to $_GET & $_POST, and is often forgotten by inexperienced developers. But there are also many other ways that users can manipulate variables used in a SQL query through cookies or even uploaded files (filenames). The only real protection is to use prepared statements everywhere consistently.
Do not use any of the mysql_* functions which have been deprecated since PHP 5.5 ,but rather use PDO, as it allows you to use other servers than MySQL out of the box. mysqli_* are still an option, but there is no real reason nowadays not to use PDO, ODBC or DBA to get real abstraction. Ideally you want to use Doctrine or Propel to get rid of writing SQL queries all together and use object-relational mapping which binds rows from the database to objects in the application.
To prevent SQL injections, one should always check & escape all user input. In PHP, this is easily forgotten due to the easy access to $_GET & $_POST, and is often forgotten by inexperienced developers. But there are also many other ways that users can manipulate variables used in a SQL query through cookies or even uploaded files (filenames). The only real protection is to use prepared statements everywhere consistently.
Do not use any of the mysql_* functions which have been deprecated since PHP 5.5 ,but rather use PDO, as it allows you to use other servers than MySQL out of the box. mysqli_* are still an option, but there is no real reason nowadays not to use PDO, ODBC or DBA to get real abstraction. Ideally you want to use Doctrine or Propel to get rid of writing SQL queries all together and use object-relational mapping which binds rows from the database to objects in the application.
7 :: Explain me what do you call the constructor of a parent class?
Just simply by parent::constructor() in an inherited class constructor.
8 :: Tell us how do you create a persistent cookie in php?
Cookies will only persist for the time you define. To do it for 1 year you can simply do:
setcookie( "cookieName", 'cookieValue', strtotime( '+1 year' ) ); //set for 1 year
setcookie( "cookieName", 'cookieValue', strtotime( '+1 year' ) ); //set for 1 year
9 :: Tell me are objects in PHP 5+ passed by value or reference?
This is a tricky question. To understand it, you need to understand how objects are instantiated.
10 :: Please explain what is the main problem in comparison of floating point numbers?
Explain the starship operator and why it returns the given output
echo 1.5 <=> 2.5; // -1
echo "a" <=> "a"; // 0
echo [1, 2, 3] <=> [1, 2, 4]; // -1
echo 1.5 <=> 2.5; // -1
echo "a" <=> "a"; // 0
echo [1, 2, 3] <=> [1, 2, 4]; // -1
11 :: Do you know what is CodeIgniter?
Codeigniter is an open source framework for web application. It is used to develop websites on PHP. It is loosely based on MVC pattern, and it is easy to use compare to other PHP framework.
12 :: Please explain what are the security parameter for XSS in CodeIgniter?
Codeigniter has got a cross-site scripting hack prevention filter. This filter either runs automatically or you can run it as per item basis, to filter all POST and COOKIE data that come across. The XSS filter will target the commonly used methods to trigger JavaScript or other types of code that attempt to hijack cookies or other malicious activity. If it detects any suspicious thing or anything disallowed is encountered, it will convert the data to character entities.
13 :: Do you know how can we get the IP address of the client?
This question might show you how playful and creative the candidate is because there are many options. $_SERVER["REMOTE_ADDR"]; is the easiest solution, but the candidate can write x line scripts for this question.
14 :: Explain me what are getters and setters and why are they important?
Getters and setters are methods used to declare or obtain the values of variables, usually private ones. They are important because it allows for a central location that is able to handle data prior to declaring it or returning it to the developer. Within a getter or setter one is able to consistently handle data that will eventually be passed into a variable or additional functions. An example of this would be a user’s name. If a setter is not being used and the developer is just declaring the $userName variable by hand, you could end up with results as such: "kevin", "KEVIN", "KeViN", "", etc. With a setter, the developer can not only adjust the value, for example, ucfirst($userName), but can also handle situations where the data is not valid such as the example where "" is passed. The same applies to a getter – when the data is being returned, it can be modifyed the results to include strtoupper($userName) for proper formatting further up the chain.
This is important for any developer who is looking to enter a team-based / application development job to know. Getters and setters are often used when dealing with objects, especially ones that will end up in a database or other storage medium. Because PHP is commonly used to build web applications, developers will run across getters and setters in more advanced environments. They are extremely powerful yet not talked about very much. It is impressive if a developer shows that he/she knows what they are and how to use them early on.
This is important for any developer who is looking to enter a team-based / application development job to know. Getters and setters are often used when dealing with objects, especially ones that will end up in a database or other storage medium. Because PHP is commonly used to build web applications, developers will run across getters and setters in more advanced environments. They are extremely powerful yet not talked about very much. It is impressive if a developer shows that he/she knows what they are and how to use them early on.
15 :: Do you know what PSR Standards do you follow? Why would you follow a PSR standard?
One should folow a PSR because coding standards often vary between developers and companies. This can cause issues when reviewing or fixing another developer’s code and finding a code structure that is different from yours. A PSR standard can help streamline the expectations of how the code should look, thus cutting down confusion and in some cases, syntax errors.
16 :: Tell me can you use include ("abc.php") two or more times?
Yes, you can include it multiple times like this.
17 :: Tell me what is the difference between $var and $$var?
$$var sets the value of $var as a variable.
18 :: Explain me the differences in comparison of variable values?
PHP defined 2 types of comparison: == and ===. Guess yourself what will be the result of each line:
☛ var_dump(1 == TRUE);
☛ var_dump(1.0 == TRUE);
☛ var_dump(1 === TRUE);
☛ var_dump(1 == TRUE);
☛ var_dump(1.0 == TRUE);
☛ var_dump(1 === TRUE);
19 :: Tell me what do you mean by having PHP as whitespace insensitive?
PHP whitespace insensitive means that it almost never matters how many whitespace characters you have in a row.
20 :: Do you know in a PHP class, what are the three visibility keywords of a property or method?
public, private, protected
21 :: Tell me why would you use === instead of ==?
If you would want to check for a certain type, like an integer or boolean, the === will do that exactly like one would expect from a strongly typed language, while == would convert the data temporarily and try to match both operand’s types. The identity operator (===) also performs better as a result of not having to deal with type conversion. Especially when checking variables for true/false, one should avoid using == as this would also take into account 0/1 or other similar representation.
22 :: Tell me the value of the variable input is a string 1,2,3,4,5,6,7.
How would you get the sum of the integers contained inside input?
<?php
echo array_sum(explode(',',$input));
?>
The explode function is one of the most used functions in PHP, so it’s important to know if the developer knows this function. There is no unique answer to this question, but the answer must be similar to this one.
echo array_sum(explode(',',$input));
?>
The explode function is one of the most used functions in PHP, so it’s important to know if the developer knows this function. There is no unique answer to this question, but the answer must be similar to this one.
23 :: Do you know what is the difference between GET and POST?
☛ GET displays the submitted data as part of the URL, during POST this information is not shown as it’s encoded in the request.
☛ GET can handle a maximum of 2048 characters, POST has no such restrictions.
☛ GET allows only ASCII data, POST has no restrictions, binary data are also allowed.
Normally GET is used to retrieve data while POST to insert and update.
Understanding the fundamentals of the HTTP protocol is very important to have for a PHP developer, and the differences between GET and POST are an essential part of it.
☛ GET can handle a maximum of 2048 characters, POST has no such restrictions.
☛ GET allows only ASCII data, POST has no restrictions, binary data are also allowed.
Normally GET is used to retrieve data while POST to insert and update.
Understanding the fundamentals of the HTTP protocol is very important to have for a PHP developer, and the differences between GET and POST are an essential part of it.
24 :: Tell me why is there a need to configure the URL routes?
Changing the URL routes has some benefits like
☛ From SEO point of view, to make URL SEO friendly and get more user visits
☛ Hide some URL element such as a function name, controller name, etc. from the users for security reasons
☛ Provide different functionality to particular parts of a system
☛ From SEO point of view, to make URL SEO friendly and get more user visits
☛ Hide some URL element such as a function name, controller name, etc. from the users for security reasons
☛ Provide different functionality to particular parts of a system
25 :: Tell me what are hooks in CodeIgniter?
Codeigniter’s hooks feature provides a way to change the inner working of the framework without hacking the core files. In other word, hooks allow you to execute a script with a particular path within the Codeigniter. Usually, it is defined in application/config/hooks.php file.